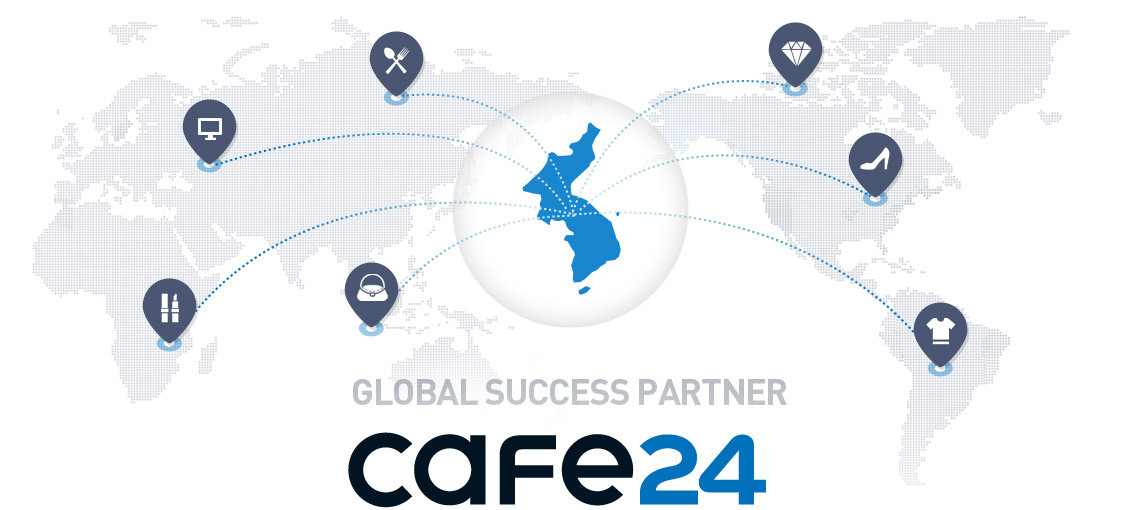
2022.07.27
[Python, API] Cafe24를 통해 알아보는 OAuth 2.0 기반의 API 호출 방법 (Part 1)
[Python, API] Cafe24를 통해 알아보는 OAuth 2.0 기반의 API 호출 방법 (Part 2) (현재 글)
이전 글에서는 인증 코드를 발급받는 것까지 진행하였습니다.
이번 글에서는 액세스 토큰을 발급받는 것과 액세스 토큰을 이용하여 API를 호출하는 것까지 진행해보겠습니다.
Cafe24 개발자센터 (API)
https://developers.cafe24.com/
카페24 개발자센터
카페24 오픈 API를 활용해 쇼핑몰 운영자를 대상으로 앱 비즈니스 기회를 창출할 수 있습니다.
developers.cafe24.com
인증 코드로 액세스 토큰 발급받기
앞서 발급받은 인증 코드를 이용하여 액세스 토큰 발급 요청을 하겠습니다.
python을 이용하여 액세스 토큰에 Request하는 형식은 다음과 같습니다.
import requests
url = "https://{mallid}.cafe24api.com/api/v2/oauth/token"
payload = "grant_type=authorization_code&code={code}&redirect_uri={redirect_uri}"
headers = {
"Authorization": "Basic {base64_encode({client_id}:{client_secret})}",
"Content-Type": "application/x-www-form-urlencoded"
}
response = requests.request("POST", url, data=payload, headers=headers)
print(response.text)
파라미터 | 설명 | |
mallid | 쇼핑몰ID를 입력하면 됨. | |
client_id | 앱 생성시 발급받은 클라이언트 ID를 입력하면 됨. | |
client_secret | 앱 생성시 받은 시크릿 키를 입력하면 됨. | |
code | 발급받은 인증 코드를 입력하면 됨. | |
redirect_uri | 앱 생성시 등록하였던 Redirect URI를 입력하면 됨. |
이때 클라이언트 ID와 시크릿 키를 base64로 encoding한 결괏값을 'Authorization'이라는 헤더에 삽입해야 합니다.
base64로 encoding하기 위해 제가 주로 이용하는 방법은 크게 두 가지가 있습니다.
- base64encode 사이트를 이용하기
- python에서 base64 라이브러리를 사용하기
첫 번째로, base64encode 사이트를 이용하는 방법부터 소개하겠습니다.
base64encode
Base64 Decode and Encode - Online
Decode from Base64 format or encode into it with various advanced options. Our site has an easy to use online tool to convert your data.
www.base64decode.org
사이트에서 '{client_id}:{client_secret}' 값을 입력 후 'ENCODE' 버튼을 눌러줍니다.
그러면 다음과 같이 base64로 encoding한 결괏값을 얻을 수 있습니다.
두 번째로, python에서 base64 라이브러리를 사용하는 방법을 알려드리겠습니다.
import requests
import base64
authentication_code = 'x'
client_id = 'x'
client_secret_key = 'x'
# base64로 encoding하기 위한 코드
id_secret = client_id + ":" + client_secret_key
base64_encoded = base64.b64encode(id_secret.encode()).decode()
url = "https://{mallid}.cafe24api.com/api/v2/oauth/token"
payload = "grant_type=authorization_code&code=" + authentication_code + "&redirect_uri={redirect_uri}"
headers = {
"Authorization": "Basic " + base64_encoded,
"Content-Type": "application/x-www-form-urlencoded"
}
response = requests.request("POST", url, data=payload, headers=headers)
print(response.text)
authentication_code, client_id, client_secret_key만 설정하면 바로 액세스 토큰을 발급받을 수 있도록 제가 직접 작성한 코드입니다.
코드를 간단하게 설명하자면, base64 라이브러리 안의 b64encode 함수를 이용하여 클라이언트 ID와 시크릿 키를 base64로 encoding 해주는 것입니다.
파라미터를 채워넣고 authentication_code, client_id, client_secret_key에 알맞은 값을 입력한 후, 코드를 실행시켜 보겠습니다.
{
"access_token": "0iqR5nM5EJIq..........",
"expires_at": "2022-07-26T14:00:00.000",
"refresh_token": "JeTJ7XpnFC0P..........",
"refresh_token_expires_at": "2022-08-08T12:00:00.000",
"client_id": "BrIfqEKoPxeE..........",
"mall_id": "yourmall",
"user_id": "test",
"scopes": [
"mall.read_order",
"mall.read_product",
"mall.read_store",
"...etc...",
],
"issued_at": "2022-07-26T12:00:00.000"
}
그러면 다음과 같은 형식의 Response를 반환합니다.
여기서 'access_token'의 값만 추출하여 API 호출에 이용하면 됩니다.
리프레시 토큰으로 액세스 토큰 발급받기
그런데 액세스 토큰은 유효기간이 2시간밖에 되지 않습니다.
그래서 2시간마다 재발급을 받아야 하는데 위와 같은 과정을 다시 거쳐서 발급받자니 너무 귀찮습니다.
이러한 상황에서 액세스 토큰을 쉽게 재발급받을 수 있도록 도와주는 것이 리프레시 토큰입니다.
이번에는 유효기간이 2주인 리프레시 토큰을 이용하여 액세스 토큰을 다시 발급받을 수 있는 방법을 알아보도록 하겠습니다.
python에서 리프레시 토큰을 이용하여 다시 액세스 토큰을 Request하는 형식은 다음과 같습니다.
import requests
url = "https://{mallid}.cafe24api.com/api/v2/oauth/token"
payload = "grant_type=refresh_token&refresh_token={refresh_token}"
headers = {
"Authorization": "Basic {base64_encode({client_id}:{client_secret})}",
"Content-Type": "application/x-www-form-urlencoded"
}
response = requests.request("POST", url, data=payload, headers=headers)
print(response.text)
아래는 refresh_token, client_id, client_secret_key만 설정하면 바로 액세스 토큰을 발급받을 수 있도록 제가 직접 작성한 코드입니다.
import requests
import base64
refresh_token = 'x'
client_id = 'x'
client_secret_key = 'x'
# base64로 encoding하기 위한 코드
id_secret = client_id + ":" + client_secret_key
base64_encoded = base64.b64encode(id_secret.encode()).decode()
url = "https://{mallid}.cafe24api.com/api/v2/oauth/token"
payload = "grant_type=refresh_token&refresh_token=" + refresh_token
headers = {
"Authorization": "Basic " + base64_encoded,
"Content-Type": "application/x-www-form-urlencoded"
}
response = requests.request("POST", url, data=payload, headers=headers)
print(response.text)
값을 넣고 실행시키면,
{
"access_token": "0iqR5nM5EJIq..........",
"expires_at": "2022-07-26T14:00:00.000",
"refresh_token": "JeTJ7XpnFC0P..........",
"refresh_token_expires_at": "2022-08-08T12:00:00.000",
"client_id": "BrIfqEKoPxeE..........",
"mall_id": "yourmall",
"user_id": "test",
"scopes": [
"mall.read_order",
"mall.read_product",
"mall.read_store",
"...etc...",
],
"issued_at": "2022-07-26T12:00:00.000"
}
아까와 같은 형식의 Response를 반환하는 것을 확인할 수 있습니다.
아까와 마찬가지로 여기서 'access_token'의 값만 추출하여 API 호출에 이용하면 됩니다.
액세스 토큰으로 API 호출하기
마지막으로 액세스 토큰을 이용하여 API를 호출하는 방법을 알아보겠습니다.
일단 가장 기본적인 상품 목록 데이터를 추출해보겠습니다.
python에서 API를 호출하기 위해 Request하는 형식은 다음과 같습니다.
import requests
url = "https://{mallid}.cafe24api.com/api/v2/admin/products"
headers = {
'Authorization': "Bearer {access_token}",
'Content-Type': "application/json",
'X-Cafe24-Api-Version': "{version}"
}
response = requests.request("GET", url, headers=headers)
print(response.text)
{access_token}에는 방급 발급받은 액세스 토큰 값을 넣고,
{version}에는 앱 생성시 설정한 버전 값을 입력하면 됩니다.
입력 후 코드를 실행하면 다음과 같은 형식의 Response를 반환합니다.
{
"products": [
{
"shop_no": 1,
"product_no": 24,
"product_code": "P000000X",
"custom_product_code": "",
"product_name": "iPhone X",
"eng_product_name": "iPhone Ten",
"supply_product_name": "iphone A1865 fdd lte",
"internal_product_name": "Sample Internal product name",
"model_name": "A1865",
"price_excluding_tax": "1000.00",
"price": "1100.00",
"retail_price": "0.00",
"supply_price": "9000.00",
"display": "T",
"selling": "F",
"product_condition": "U",
"product_used_month": 2,
.
.
.
]
}
]
}
성공적으로 상품 목록 데이터를 추출하였습니다!
이로써 OAuth 2.0 기반의 API 호출 방법을 모두 알아볼 수 있었습니다.
- [Python, API] Cafe24를 통해 알아보는 OAuth 2.0 기반의 API 호출 방법 (Part 1)
- [Python, API] Cafe24를 통해 알아보는 OAuth 2.0 기반의 API 호출 방법 (Part 2) (현재 글)